DEWI
By Shawn Pinciara and Romeo Gabriele
Github repo: https://github.com/shawnpinciara/dewi

Introduction:
Wind instruments are physically limited of having the holes in strict positions to achieve the sound of the instrument. This limitation was not overcome with electronic wind instruments, as the current standard in the market is still to replicate the acoustic ones.
That’s why the DEWI was born, to experiment both finger positioning and finger mapping for the notes, efficiently decreasing the number of buttons needed (the instrument could work just with 7 buttons for 4 octaves without the 4 buttons to change key).
Name and Fundamental Idea:
The name dEWI stands for Diatonic Ewi. This layout is part of the discussion with the musician Garaggio (Romeo Gabriele) who helped to create an organic layout which helps to pass from certain notes to other easily.
In jazz music, after studying functional harmony and non, as a musician you’ll understand how to play unconsciously. You don’t have to know every song’s chords. For istance, you can use pentatonics, widely used in blues, as a pattern of whatever five-group notes: so, if there’s an alteration in a modal scale, you would include it in a new pentatonic. Of course, the idea works in the opposite direction too, which is choosing a pentatonic scale starting from another I grade compared to key’s song, including some note altered in a clever way.
So, why not trying the feeling of being able to control it in a
performative way We created a layout that let you play every note with one hand only. This allows to choose the played note with the left hand , and the key of your pentatonic scale with the right one - as mentioned in the name “dEWI”, in a diatonic way.
Here a physical layout representation of buttons pressing combinations.

Playing Functioning:
The layout is tailored to the pentatonic scale, thanks to several details. In fact, after the III grade you find the V one, in a successive button press, instead of IV, useless in the pentatonic approach. Same with the passage from VI to VII, that is more complex than the passage from VI to VII, since it’s more useful.

We designed the fourth button (used with the pinky) for alterations, only in one direction: sharps (#). The only exception is found on the DO8 combination (I in the higher octave), so adding the Sharp to that actually allows you to play the III of the higher octave, allowing to play smarter the iconic minor solo loop passage VII-I-III. Of course, adapting your playing to a minor song requires you to shift to the relative major key with your right hand.
Note that notes are changed by the left hand both by pressing and leaving the buttons, while for the key (right hand) only press down are detected (so if you remove your hand it won’t return to the C key) leaving the possibility to play another instrument with the right hand! There is no blank combination of the right hand (apart from when you start to play) so selecting the C key have a precise buttons’ combination.

The 4 notes buttons have a curve shape to accomodate the use of the left hand, while the 4 keys buttons have the exact same layout but mirrored.
At the top of the instrument there is a peculiar squared mouthpiece with a tube in it; a connector cloud be added to insert a standard sax/woodwinds mouthpiece.
Finally, on the back, three button are dedicated to the octave selection. The two left-thumbed switches allow to select the higher and lower octave from the center one (C4), while the right-thumbed switch (only on press-down) shifts the center of the octave from C4 to C2. We call it the “bass mode”.
Implementation:
Hardware:

All mounted on a prototype board, the form factor of the circuit is about the size of a credit card!
To have a minimal setup just a few components are needed to make the DEWI work:
- Microcontroller (Arduino Pro Micro and ESP32 both tested)
- hx710b (breath module based on the sensor MPS20N0040D-D)
And one additional component to make the buttons capacitive and easier to scan (as the module use i2c):
- MPR121
Later in the project the ESP32 (that was used because of the MIDI Bluetooth capabilities but do not have USB MIDI capabilities) was swapped for the Arduino Pro Micro instead (that has USB MIDI), MIDI Bluetooth has been removed from the project because of the unreliability of connection with Apple devices, but it still could be used in a live environment as the delays are pretty low (not noticeable in my project).
A 5din MIDI connector was soldered onto the board but not implemented in the code (it was left open for future implementations).
Software:
Considering the use of the MPR121 I2C touch sensor, the whole program is based around the concept of busy waiting on the sensor reading and then processing both the touch sensor and the breath sensor readings to output the correct midi values.
The MPR121 output a 12bit value in which every bit corresponds to a key state, so the first stage was to make masks accordingly to filter out the 3 categories of keys: notes,keys,octave
The 4 bit value (the note played) is then converted to a decimal number which represent the index of an array where all midi values are stored.
const uint16_t mask_key = 0b0000000011110000;
const uint16_t mask_note = 0b0000000000001111;
const uint16_t mask_octave = 0b0000111100000000;
const uint16_t mask_cc = 0b0000100000000000;
The conversion from the sensor reading to the note midi goes as follows (example for the note D):
0001 (binary) → 1 (decimal) → arr[1] = 50 (MIDI value)
const int noteArray[16] = {0,2,11,4,12,9,5,7,1,3,0,0,16,10,6,8};
const int keyArray[16] = {0,2,11,4,0,9,5,7,1,3,0,0,16,10,6,8};
const int octaveArray[16] = {0,1,-1,0,0,0,0,0,-2,-1,-3,0,0,0,0,0};
Having then also the value of the octave (token from the octave bits) the final current note can be calculated as follow:
currentNote = ((octave+octaveArray[currentOctave])*12)+
(noteArray[mpr121 & mask_note]+keyArray[currentKey]);
Having the the note to play, there are 3 main states in which the user can send or release a note, which are:
- Starting to blow
- Has to operate just once every time there is a new blow
if (breath > threshold_bottom) { //a threashold for blowing is put
velocity = map(breath,threshold_bottom,threshold_top,40,127);
lastKey = currentKey;
mpr121 = mpr.touched(); //sensor reading
//bits masking
currentKey = (mpr121 & mask_key)>>4;
currentOctave = (mpr121 & mask_octave)>>8;
currentNote = ((octave+octaveArray[currentOctave])*12)+(noteArray[mpr121 & mask_note]+keyArray[currentKey]);
if (currentKey == 0) { //*1 see note below
currentKey = lastKey; }
if (breathAttack) {
breathAttack=false; //debounce
breathRelease = true; //when the blow will be released
noteOn(currentNote, velocity, 1); // Send a MIDI note
...- *1: This particular case is due to the fact that the first key can be played without pushing any button (so 0000 key bits) but while playing it is better to let the key remain even while removing the hand from the instrument (so without changing the note played to the first note of the scale) to let the user possibly play another instrument too without changing key
- Changing the note while still blowing
- As busy-waiting is used, every time the loop cycle, there’s the need to check if the last note reading is equal to the current reading not to send the same note more than once (because Control Change is handled differently)
- A CC in sent as the after-touch, effectively modulating the velocity of the note while still holding that note
} else {
if (currentNote != lastNote) {
noteOff(lastNote,velocity,1);
lastNote = currentNote;
noteOn(currentNote, velocity, 1); // Send a MIDI note
} else {
controlChange(velocity,11,1); }}
- Not blowing anymore
if (breath > threshold_bottom) {
//there is other code here but not useful for this explanation
} else {
if (breathRelease==true) { //this is enabled from when you start to blow
breathAttack=true;
breathRelease=false;
noteOff(lastNote,velocity,1); }}
Design:
The instrument was based around the concept of having the two hands parallel to each other and not in a linear way (opposite to almost all the wind instruments).
The shape of it was inspired by both computer keyboards, old casio synths (with a really simple rectangular shape) held vertically and 4 holes ocarinas, in which the hands are held parallel to each other; The mouthpiece is then added as an extension of one side of the rectangle . The capacitive buttons are located on a lower plane where a space to position the hands is formed.
As the manufacturing process would be a bit too long with the technology I currently have (only a 3d printer) a wooden (with a 3d printed white mouthpiece) based prototype was built instead.
Improvements:
Tests:
Here’s a vst on a daw controlled by the DEWI:
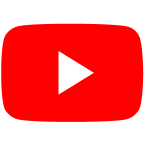
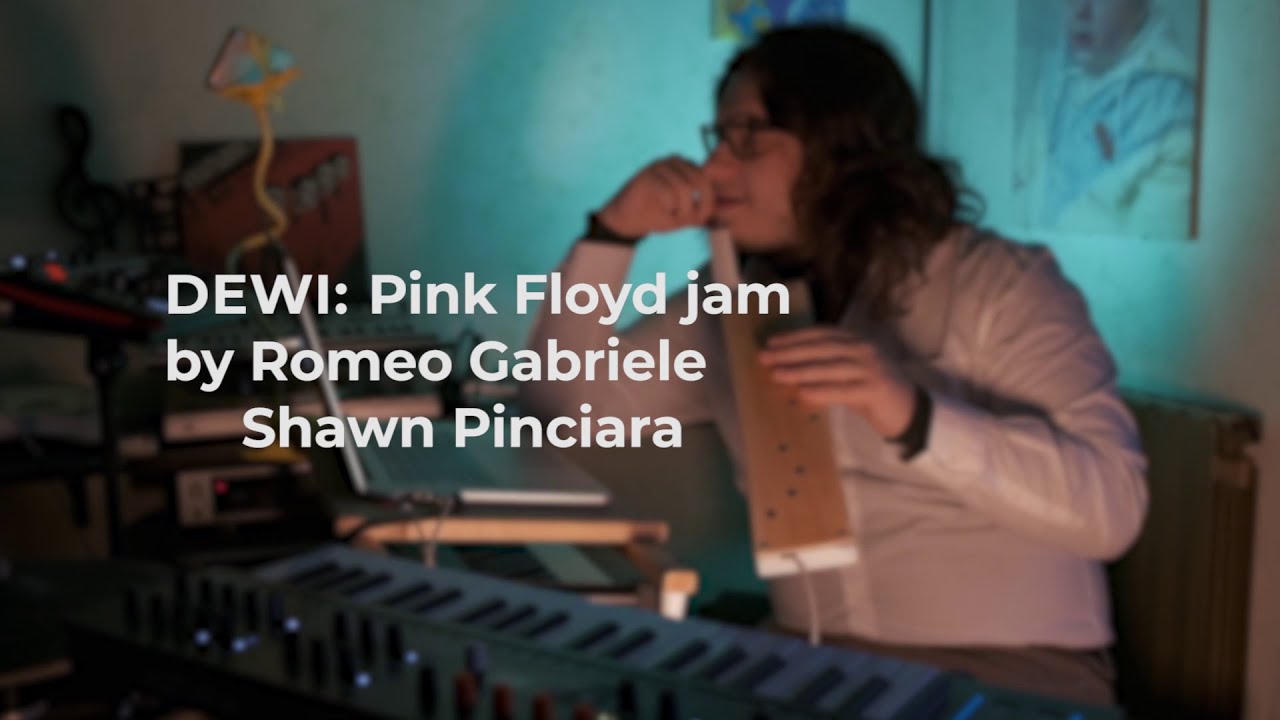
As you can see if the player is not really precise while striking more buttons at the exact same moment, the istrument will capture all the notes in between too. This type of jitter could be reduced by adding a small delay in between each reading of the buttons, it should not compromise the reactiveness of the instrument though.
Future implementations:
Thinking about expanding the instrument capabilities, the first idea would be to add a midi 5 din support, to control other older synths.
Other than that, the DEWI could also be an autonomous monophonic (and 2 drone polyphonic too) synth, by using on of the popular DSP for microcontrollers (such as FAUST for the ESP32/Teensy or Mozzi for the other microcontrollers) and on the hardware side adding a simple PWM out (with an rc filter) or interfacing it with an I2C audio board.
Functionality wise, more sensors (such as a gyro, bending potentiometer/soft linear pots) could be added to increment the overall expressiveness of the instrument, by adding controllable vibrato/pitch-bend or other after-touch controls.